Visualization tool
The QUANTEEC Visualization tool is an optional add-on to display simple QUANTEEC metrics for a player, such as:
- The quantity of data downloaded from CDN and P2P
- The quantity of data sent in P2P
- The quantity of energy saved by sending or receiving data through P2P
The Visualization tool can be installed directly inside your Android app.
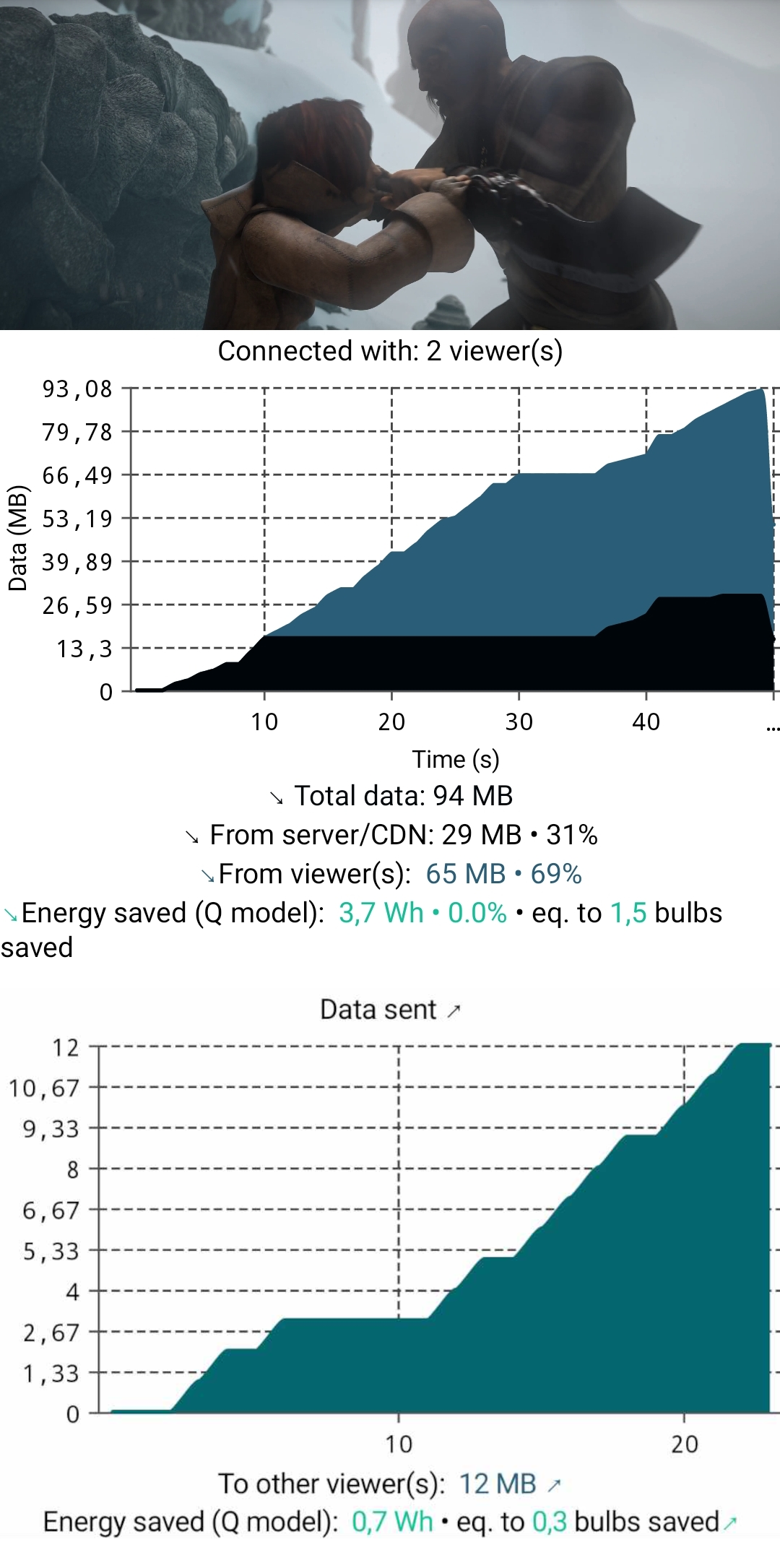
Installing the QUANTEEC Visualization tool as a dependency
1- Declare the Maven repository host on jitpack.io
.
The QUANTEEC Maven repository is also required. However, normally, it has already been declared for the integration of QUANTEEC plugin into the Android player.
repositories {
...
maven {
url 'https://maven.quanteec.com/repository/internal/'
credentials {
username "username"
password "password"
}
authentication {
basic(BasicAuthentication)
}
maven { url 'https://jitpack.io' }
}
}
To obtain your credentials for QUANTEEC Maven repository, please contact us by mail.
2- Add the following dependency to your build.gradle
file:
repositories {
dependencies {
...
implementation "com.quanteec:quanteecExoVisualize:1.2.0"
}
}
Using the QUANTEEC Visualization tool
Using the tool with Jetpack Compose
Using the QUANTEEC Visualization tool with Jetpack Compose is fairly simple. All you have to do is to create an instance of the tool and display it by calling the method DisplayVisualizationTool()
, as shown in the example below:
//1. Instantiate the Visualization tool
val visuTool = QuanteecVisualize(quanteecCore)
//2. Display the Visualization tool
visuTool.DisplayVisualizationTool();
Using the Visualization tool with Views
To use the QUANTEEC Visualization tool with Android Views, you first need to add one more dependency in your gradle.build
file:
implementation("androidx.activity:activity-compose:1.7.2")
Then, create a ComposeView in order to display the Visualization tool.
<androidx.compose.ui.platform.ComposeView
android:id="@+id/visu_view"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:background = "#ffffff"/>
</LinearLayout>
In the code of your application, add the following lines to instantiate and display the Visualization tool:
//1. Instantiate the Visualization tool
QuanteecVisualize visuTool = new QuanteecVisualize(/* QuanteecCore = */ quanteecCore);
//2. Get the view
ComposeView visuView = findViewById(R.id.visu_view);
//3. Display the tool
visuTool.displayVisualizationToolFromView(visuView);